The Half-Distance Paradox. Simulation in Go
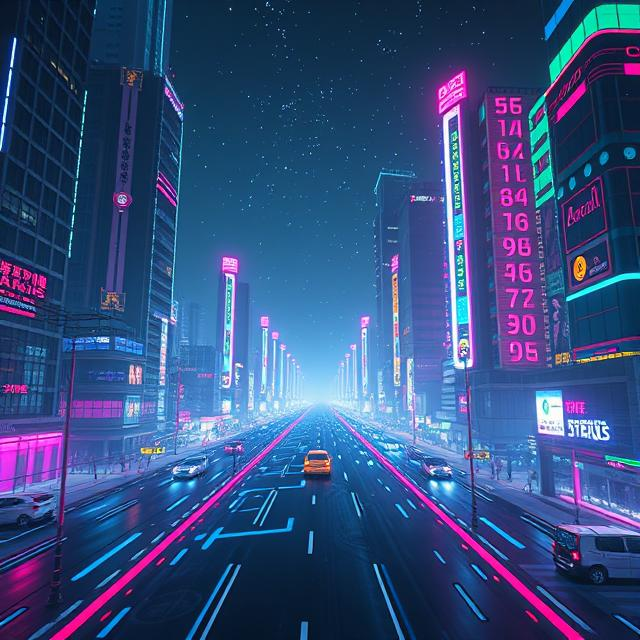
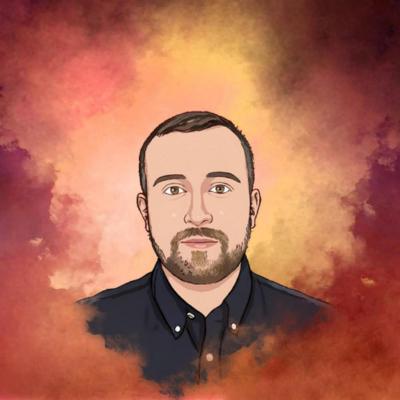
Understanding the Half-Distance Paradox
In this article, we'll explore a fascinating concept often referred to as the "half-distance paradox," using Go. This paradox comes from an idea in mathematics and philosophy, where a person or object is thought to always halve the distance between themselves and their goal, making it appear that they can never actually reach their destination. This program will simulate the concept and give us some insight into the way it behaves in computing.
Imagine you start at a specific distance from a goal, and with each step, you cover half of the remaining distance. No matter how many times you take a step, the remaining distance is always halved. It seems like you'll never quite reach the goal because you're always left with a smaller distance to travel.
How does the simulation works?
The Go program provided simulates this paradox by starting with an initial distance and repeatedly halving it. Here's how it works:
Starting with a distance, the program accepts an integer input that represents the initial distance.
Halving the distance. The program enters a loop, where each time it divides the current distance by 2.
Printing the results. It prints out the current distance after each halving, first in scientific notation and then as a human-readable floating-point number.
The loop continues until the remaining distance becomes so small that it is effectively zero due to the limits of floating-point precision.
Let’s break it down in more detail:
The code
package main
import (
"fmt"
"os"
"strconv"
)
func runParadoxSimulation(distance int) float64 {
fmt.Println("runParadoxSimulation distance", distance)
// Convert the distance to a float64 for better precision
temp := float64(distance)
// Start halving the distance
for temp > 0 {
temp = temp / 2
// Print the current distance in scientific notation
fmt.Println("each item", temp)
// Print the current distance as a floating-point number
fmt.Printf("Using %%f: %.324f\n", temp)
}
return temp
}
func main() {
// Check if the user provided a distance as an argument
if len(os.Args) < 2 {
fmt.Println("Provide an integer argument for distance.")
return
}
// Parse the distance from the command-line arguments
distance, err := strconv.Atoi(os.Args[1])
if err != nil {
fmt.Println("Unable to parse incoming arguments")
return
}
// Run the paradox simulation
result := runParadoxSimulation(distance)
// Print the last computed value
fmt.Println("last item computed", result)
}
Terminal results example (last 3 values printed)
Running in terminal go run main.go 42
. You can start with any integer value you like.
each item 1e-323
Using %f: 0.000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000010
each item 5e-324
Using %f: 0.000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000005
each item 0
Using %f: 0.000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000
The loop in runParadoxSimulation repeatedly halves the value of temp (which starts as the input distance). Each time it divides by 2, the distance gets smaller, simulating the idea of reducing the distance by half in each step.
The result is printed in both scientific notation (which shows the distance in terms of powers of 10) and as a human-readable floating-point number. The %.324f format specifier is used to print a highly precise floating-point number, emphasizing the smallness of the number as it gets closer to zero.
The loop continues until the distance becomes so small that further halving results in zero due to the limitations of floating-point precision in computers. This is where the paradox comes into play—eventually, the distance becomes so small that the computer can no longer represent it accurately, effectively reaching zero.
In real life, the paradox of halving the distance to a goal indefinitely may seem like an impossible task. But in the world of computers, floating-point numbers have a limited precision. After repeatedly halving the distance, the computer reaches a point where the number is so small that it can no longer represent it accurately. In Go, as in many other languages, this is because floating-point numbers are stored with a finite number of digits.
The smallest positive number that can be represented in Go’s float64 type is approximately 5e-324. Once the number becomes smaller than this, it effectively becomes zero, even though conceptually, it should still have some value. This behavior is a consequence of how computers handle numbers and is a practical illustration of the "half-distance" paradox.
The half-distance paradox is a great way to visualize the concept of infinity and limits. In the case of this Go program, it demonstrates how halving a number repeatedly can lead to results that are so small they fall below the precision limits of the computer, effectively reaching zero.
Understanding these limitations is important for working with floating-point arithmetic in programming. While the paradox shows that theoretically, you can keep halving forever, computers show us the practical consequences of finite precision.
The program serves as both an interesting demonstration of a philosophical concept and a lesson in how computers handle real numbers. Even though we might never physically reach the destination by halving the distance, the simulation helps us see how the "infinite" can be bounded by the practical limits of computation.
Thank you for following so far. The source code can be found here https://github.com/GeorgeCrisan/half-distance-paradox. Run it with air (the repository has config set with a default value) or pass the entry value as go run main.go 42
.