Golang, Go read JSON data from a file and create a slice of struct
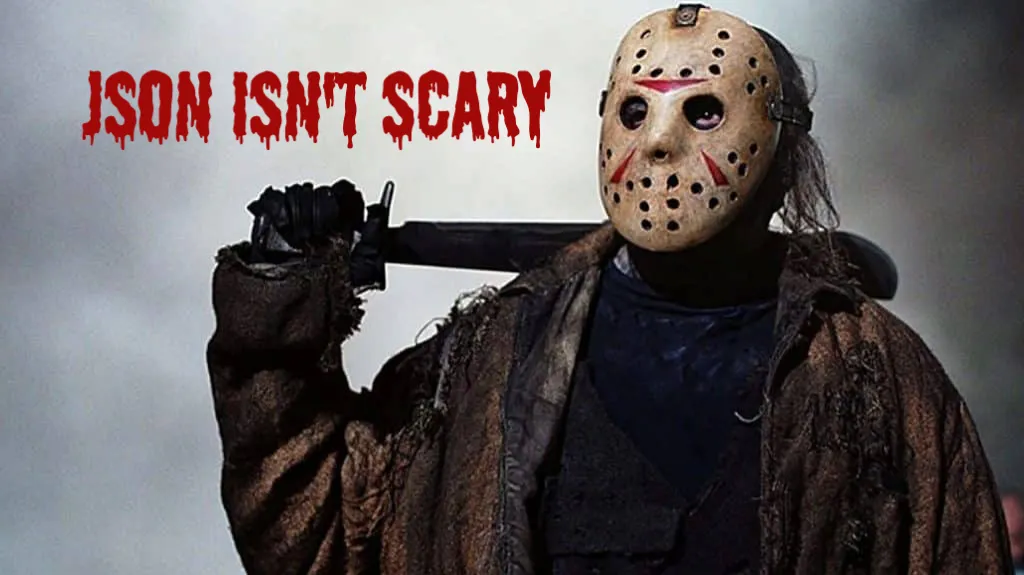
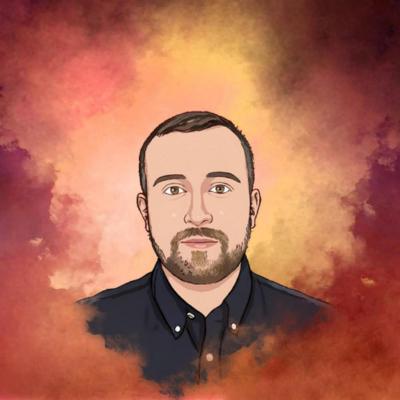
George Crisan
Posted on:
Content
Full example at https://github.com/GeorgeCrisan/go-read-json-from-file/tree/main
Save the mock data to a file on the root directory. File name people.json
.
This is the sample JSON data.
[
{
"name": "Marilyn Langley",
"gender": "female",
"email": "marilynlangley@snowpoke.com",
"bestFriends": [
{
"name": "Tanner Stewart"
},
{
"name": null
},
{
"name": "Claire Monroe"
}
],
"favoriteFruit": "strawberry"
},
{
"name": "Norma Torres",
"gender": "female",
"email": "normatorres@snowpoke.com",
"bestFriends": [
{
"name": "Kaitlin Nixon"
},
{
"name": "Freida Mason"
}
],
"favoriteFruit": "banana"
},
{
"name": "Gaines Richmond",
"gender": "male",
"email": "gainesrichmond@snowpoke.com",
"bestFriends": [
{
"name": "Sylvia Christian"
},
{
"name": "Kathie Nolan"
}
],
"favoriteFruit": "apple"
},
{
"name": "Bruce Matthews",
"gender": "male",
"email": "brucematthews@snowpoke.com",
"bestFriends": [
{
"name": "Stacy Webb"
}
],
"favoriteFruit": "apple"
},
{
"name": "Watson Long",
"gender": "male",
"email": "watsonlong@snowpoke.com",
"bestFriends": [
{
"name": "Mooney Vaughan"
},
{
"name": "Glenda Harrison"
},
{
"name": "Sawyer Decker"
}
],
"favoriteFruit": "strawberry"
},
{
"name": "Howard Carlson",
"gender": "male",
"email": "howardcarlson@snowpoke.com",
"bestFriends": [
{
"name": "Merle Kemp"
},
{
"name": "Brittany Ingram"
}
],
"favoriteFruit": "banana"
},
{
"name": "Vonda Salinas",
"gender": "female",
"email": "vondasalinas@snowpoke.com",
"bestFriends": [
{
"name": "Knapp Gay"
},
{
"name": "Bush Lott"
},
{
"name": "Elnora Warren"
}
],
"favoriteFruit": "banana"
}
]
The utils file. /utils/utils.go
Here we define the types that match the json data pretty much as schema.
The function check
is meant to aid with handling errors.
The function ReadJsonFromFile
reads the JSON file and returns the data as a slice of bytes.
Making use of Unmarshal method we map the json to the slice collection using GetPersonsCollection
.
package utils
import (
"encoding/json"
"fmt"
"os"
)
type Friend struct {
Name string `json:"name"`
}
type Person struct {
Name string `json:"name"`
Gender string
Email string
BestFriends []Friend
FavoriteFruit string
}
func check(err error) {
if err != nil {
fmt.Println("Something went wrong", err)
return
}
}
func ReadJsonFromFile(fileName string) []byte {
dataByes, err := os.ReadFile(fileName)
check(err)
return dataByes
}
func GetPersonsCollection(data []byte, withPrintSample bool) []Person {
var collection []Person
err := json.Unmarshal(data, &collection)
check(err)
if withPrintSample && len(collection) > 0 {
sample, err := json.MarshalIndent(collection[0], "", "\t")
check(err)
fmt.Printf("Here is a sample: %+v\n", string(sample))
}
return collection
}
Entry file
Here we make use of the util functions defined above.
The main file main.go
package main
import (
"fmt"
"json-playground/utils"
)
func main() {
rawData := utils.ReadJsonFromFile("people.json")
result := utils.GetPersonsCollection(rawData, true)
fmt.Printf("Result length: %v\n", len(result))
}
Hope you enjoyed.